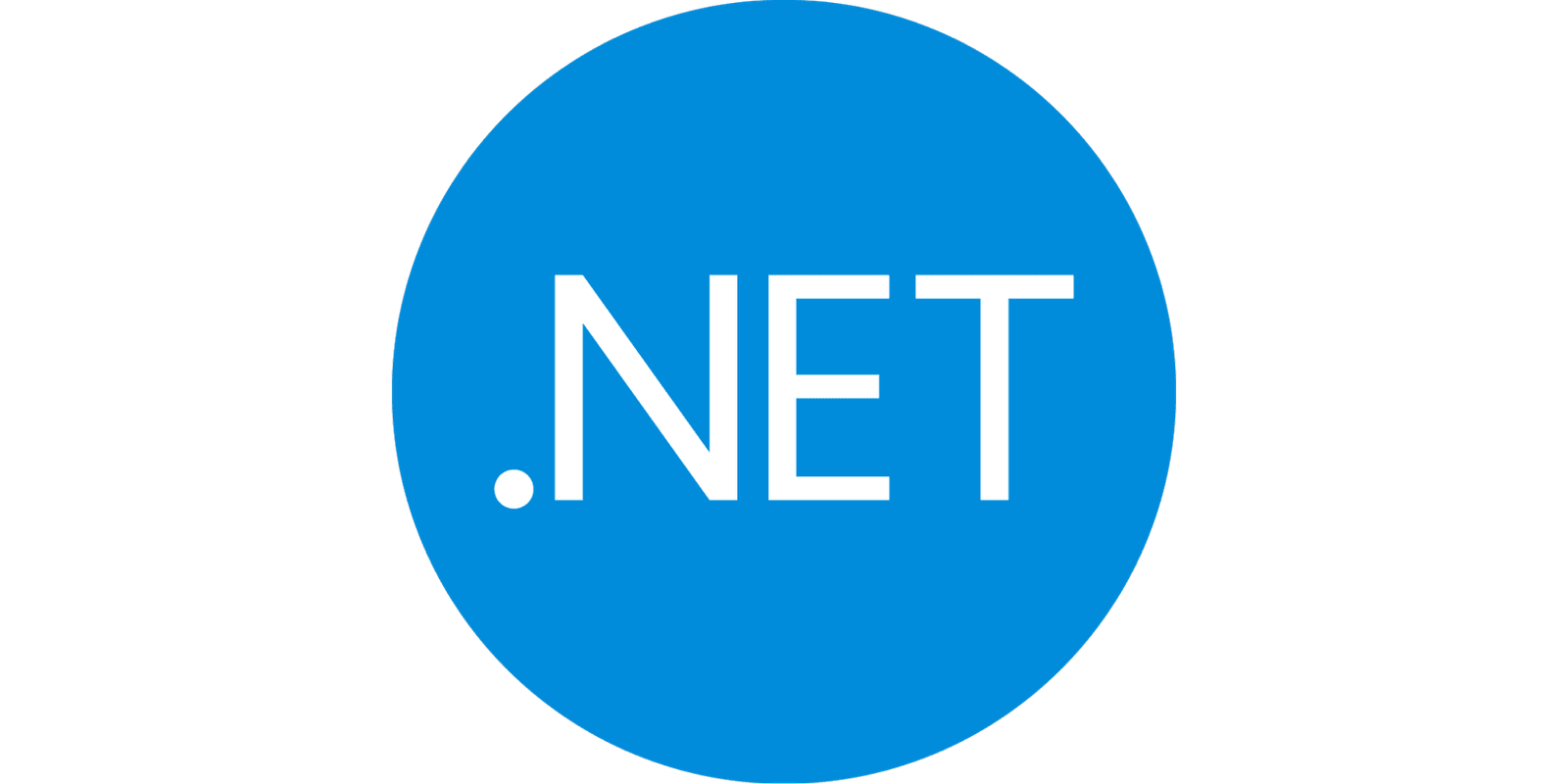
.NET
.NET is a free, cross-platform, open-source developer platform created by Microsoft for building many types of applications. With .NET, you can use multiple languages, editors, and libraries to build for web, mobile, desktop, gaming, and IoT. This guide will provide an overview of .NET's key features, installation process, and tips for effective use.
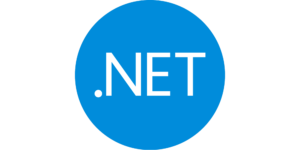
.NET: The Ultimate Guide
Key Features
- Cross-Platform: Develop applications that run on Windows, macOS, and Linux.
- Versatile Application Development: Build web, mobile, desktop, cloud, gaming, and IoT applications.
- Unified Framework: Provides a consistent programming model and APIs across various types of applications.
- Multiple Languages: Supports C#, F#, and Visual Basic.
- Rich Ecosystem: Extensive libraries, frameworks (like ASP.NET), and tools (like Visual Studio) to enhance development.
- High Performance: Optimized runtime for high performance and scalability.
- Strong Security: Built-in security features to protect applications from various threats.
- Advanced Tooling: Integrated with powerful development tools such as Visual Studio and Visual Studio Code.
- Open Source: Actively developed and maintained by a large community.
For macOS:
- Download Node.js:
- Visit the Node.js download page.
- Choose the appropriate installer for macOS (LTS or Current version) and download it.
- Install Node.js:
- Open the downloaded
.pkg
file. - Follow the setup wizard instructions to complete the installation.
- Open the downloaded
- Verify Installation:
- Open Terminal and run
node -v
to check the Node.js version. - Run
npm -v
to check the npm version.
- Open Terminal and run
For Linux:
- Download and Install Node.js:
- Open Terminal.
- Use a package manager to install Node.js. For example, on Debian-based systems:
sudo apt update
sudo apt install nodejs npm
- Verify Installation:
- Run
node -v
to check the Node.js version. - Run
npm -v
to check the npm version.
- Run
Using .NET
Creating a New Project:
- Create a Project Directory:
- Open your terminal or command prompt.
- Create a new directory and navigate into it:
mkdir mydotnetapp cd mydotnetapp
- Initialize a New Project:
- Use the .NET CLI to create a new project:
dotnet new console
- Use the .NET CLI to create a new project:
- Run the Project:
- Build and run the project:
dotnet run
- Build and run the project:
Building Web Applications:
- Create an ASP.NET Core Project:
- Use the .NET CLI to create a new ASP.NET Core web application:
dotnet new webapp -o myWebApp cd myWebApp
- Use the .NET CLI to create a new ASP.NET Core web application:
- Run the Web Application:
- Build and run the web application:
dotnet run
- Open a browser and navigate to
http://localhost:5000
to see the web application.
- Build and run the web application:
Advanced Features
Dependency Injection:
- Configuring Services:
- Register services in
Startup.cs
:public void ConfigureServices(IServiceCollection services) { services.AddTransient<IMyService, MyService>(); }
- Register services in
- Using Services:
- Inject services into your controllers or classes:
public class HomeController : Controller { private readonly IMyService _myService; public HomeController(IMyService myService) { _myService = myService; } public IActionResult Index() { var data = _myService.GetData(); return View(data); } }
- Inject services into your controllers or classes:
Entity Framework Core:
- Install Entity Framework Core:
- Add the necessary packages to your project:
dotnet add package Microsoft.EntityFrameworkCore dotnet add package Microsoft.EntityFrameworkCore.SqlServer
- Add the necessary packages to your project:
- Define a Data Context:
- Create a
DbContext
class:public class MyDbContext : DbContext { public MyDbContext(DbContextOptions<MyDbContext> options) : base(options) { } public DbSet<MyModel> MyModels { get; set; } }
- Create a
- Configure the Data Context:
- Configure the data context in
Startup.cs
:public void ConfigureServices(IServiceCollection services) { services.AddDbContext<MyDbContext>(options => options.UseSqlServer(Configuration.GetConnectionString("DefaultConnection"))); }
- Configure the data context in
- Run Migrations:
- Create and apply database migrations:
dotnet ef migrations add InitialCreate dotnet ef database update
- Create and apply database migrations:
Tips for Effective Use
- Use Visual Studio or Visual Studio Code:
- Take advantage of the powerful IDEs provided by Microsoft to enhance productivity.
- Leverage NuGet Packages:
- Explore and use various NuGet packages to add functionality to your applications.
- Install packages via the .NET CLI:
dotnet add package <PackageName>
- Follow Best Practices:
- Adhere to coding standards and best practices for maintainable and scalable code.
- Use architectural patterns like MVC for web applications.
- Regularly Update .NET:
- Keep your .NET SDK and runtimes updated to benefit from the latest features, performance improvements, and security patches.
- Utilize Built-In Security Features:
- Implement security features such as authentication, authorization, and data protection to safeguard your applications.