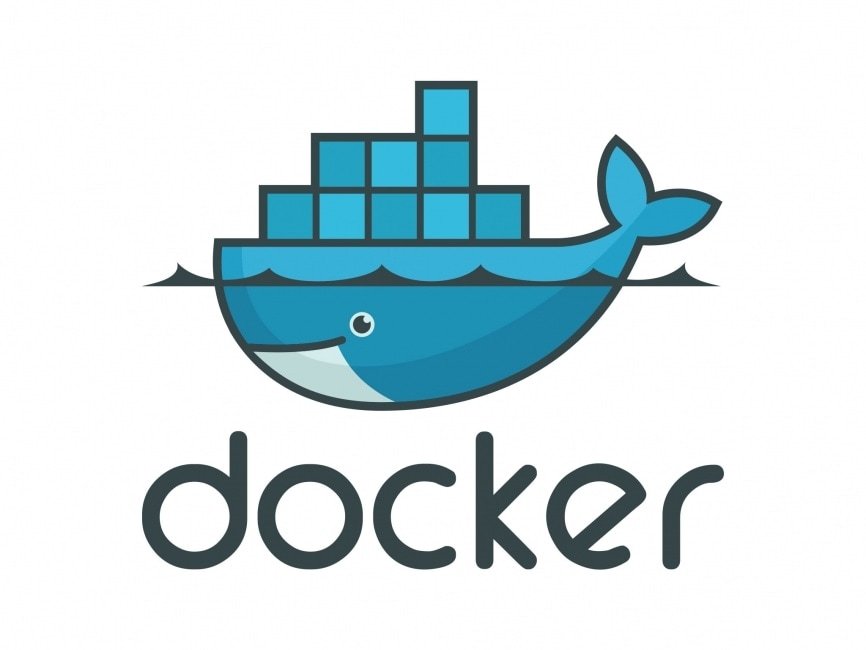
Docker
Docker is an open-source platform that automates the deployment, scaling, and management of applications using containerization. Containers are lightweight, portable units that bundle an application with all its dependencies, ensuring consistency across different environments. This guide provides an overview of Docker's key features, installation process, and tips for effective use.
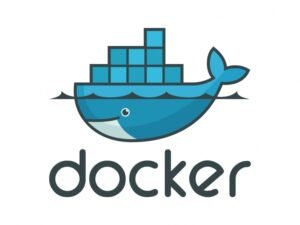
Docker: The Ultimate Guide
Key Features
- Containerization: Encapsulates applications with all their dependencies, making them portable and consistent across different environments.
- Isolation: Each container runs in its isolated environment, ensuring that different applications do not interfere with each other.
- Efficiency: Containers share the host OS kernel, making them more lightweight and faster to start than traditional virtual machines.
- Portability: Containers can run on any system that supports Docker, ensuring a consistent environment from development to production.
- Scalability: Easily scale applications horizontally by deploying multiple container instances.
- Integration: Works seamlessly with CI/CD tools and cloud platforms for automated build, test, and deployment workflows.
- Version Control: Docker images can be versioned and shared through Docker Hub or private registries.
Installation and Setup
Installing Docker
- Windows:
- Download Docker Desktop for Windows from the official Docker website.
- Run the installer and follow the setup instructions.
- After installation, launch Docker Desktop.
- macOS:
- Download Docker Desktop for Mac from the official Docker website.
- Run the installer and follow the setup instructions.
- After installation, launch Docker Desktop.
- Linux:
- Open your terminal and run the following commands:
sudo apt-get update
sudo apt-get install -y \
apt-transport-https \
ca-certificates \
curl \
gnupg-agent \
software-properties-common
curl -fsSL https://download.docker.com/linux/ubuntu/gpg | sudo apt-key add -
sudo add-apt-repository \
"deb [arch=amd64] https://download.docker.com/linux/ubuntu \
$(lsb_release -cs) \
stable"
sudo apt-get update
sudo apt-get install -y docker-ce docker-ce-cli containerd.io
sudo usermod -aG docker $USER
- Log out and log back in to apply the changes.
- Open your terminal and run the following commands:
Verifying Installation
- Verify that Docker is installed correctly by running:
docker --version
- Run a test container:
docker run hello-world
Basic Concepts
Dockerfile
- Creating a Dockerfile:
- A Dockerfile is a text file that contains instructions for building a Docker image.
- Example Dockerfile:
FROM node:14
WORKDIR /app
COPY . .
RUN npm install
EXPOSE 3000
CMD ["node", "app.js"]
Building and Running Containers
- Build an Image:
- Use the
docker build
command to create an image from a Dockerfile:docker build -t my-node-app .
- Use the
- Run a Container:
- Use the
docker run
command to start a container from an image:docker run -d -p 3000:3000 my-node-app
- Use the
- List Containers:
- View running containers using:
docker ps
- View running containers using:
- Stop a Container:
- Stop a running container using:
docker stop <container_id>
- Stop a running container using:
Advanced Features
Docker Compose
- Installing Docker Compose:
- Docker Compose is included with Docker Desktop on Windows and macOS. For Linux, install it using:
sudo curl -L "https://github.com/docker/compose/releases/download/1.29.2/docker-compose-$(uname -s)-$(uname -m)" -o /usr/local/bin/docker-compose
sudo chmod +x /usr/local/bin/docker-compose
- Docker Compose is included with Docker Desktop on Windows and macOS. For Linux, install it using:
- Creating a docker-compose.yml File:
- Define multi-container applications using a
docker-compose.yml
file:yaml
version: '3'
services:
web:
image: my-node-app
ports:
- "3000:3000"
redis:
image: "redis:alpine"
- Define multi-container applications using a
- Using Docker Compose:
- Start all services defined in the
docker-compose.yml
file:docker-compose up
- Start all services defined in the
- Stopping Services:
- Stop all running services:
docker-compose down
- Stop all running services:
Docker Swarm
- Initializing Swarm Mode:
- Initialize a new Swarm cluster:
docker swarm init
- Initialize a new Swarm cluster:
- Creating a Service:
- Deploy a service to the Swarm:
docker service create --name my-web-app -p 80:80 my-node-app
- Deploy a service to the Swarm:
- Scaling Services:
- Scale the number of service replicas:
docker service scale my-web-app=3
- Scale the number of service replicas:
Managing Images and Containers
Docker Hub
- Pushing Images to Docker Hub:
- Log in to Docker Hub:
docker login
- Tag and push your image:
docker tag my-node-app username/my-node-app
docker push username/my-node-app
- Log in to Docker Hub:
- Pulling Images from Docker Hub:
- Pull an image from Docker Hub:
docker pull username/my-node-app
- Pull an image from Docker Hub:
Docker Volumes
- Creating Volumes:
- Create a volume to persist data:
docker volume create my-volume
- Create a volume to persist data:
- Mounting Volumes:
- Mount a volume to a container:
docker run -d -p 3000:3000 -v my-volume:/app/data my-node-app
- Mount a volume to a container:
Security Best Practices
- Run Containers as Non-Root User:
- Modify your Dockerfile to use a non-root user:
FROM node:14
USER node
- Modify your Dockerfile to use a non-root user:
- Use Official Images:
- Use official images from Docker Hub to ensure you’re using secure and well-maintained software.
- Regular Updates:
- Keep Docker and your base images up-to-date to mitigate vulnerabilities.
- Limit Resource Usage:
- Use resource limits to prevent a single container from consuming too many resources:
docker run -d --cpus="1.5" --memory="512m" my-node-app
- Use resource limits to prevent a single container from consuming too many resources:
Tips for Effective Use
- Leverage Docker Compose for Development:
- Use Docker Compose to define and manage multi-container applications, making it easier to set up development environments.
- Use Multi-Stage Builds:
- Optimize your Dockerfile with multi-stage builds to reduce image size and improve security.
- Monitor Containers:
- Use monitoring tools like Prometheus, Grafana, and Docker’s built-in stats command to keep an eye on container performance.
- Automate with CI/CD:
- Integrate Docker with CI/CD pipelines using tools like GitHub Actions, Jenkins, or GitLab CI to automate building, testing, and deploying your containers.
Conclusion
Docker revolutionizes the way applications are built, shipped, and run. By leveraging containerization, Docker ensures consistency across different environments, making development and deployment more efficient. This guide covers the essential aspects of Docker, from installation to advanced features like Docker Compose and Swarm. By following best practices and effectively utilizing Docker’s features, you can streamline your development workflow and deploy scalable, reliable applications. Explore Docker’s capabilities, integrate with your CI/CD pipelines, and embrace the power of containerization to enhance your software development and deployment processes.