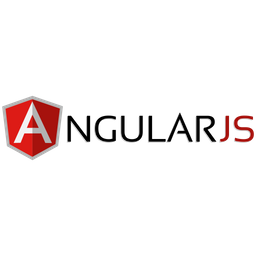
Angular JS
AngularJS is an open-source JavaScript framework developed by Google for building dynamic single-page applications (SPAs). It extends HTML with additional attributes and binds data to HTML with powerful directives. This guide provides an overview of AngularJS's key features, installation process, and tips for effective use.
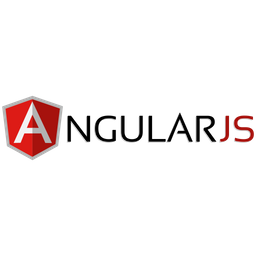
Angular JS: The Ultimate Guide
Key Features
- Two-Way Data Binding: Automatically synchronizes data between the model and the view components.
- MVC Architecture: Separates application logic, data, and presentation, making it easier to develop and test.
- Directives: Extend HTML with custom attributes and elements to build reusable components.
- Dependency Injection: Facilitates better organization and easier testing by injecting dependencies into components.
- Templates: Combines declarative HTML templates with AngularJS’s data-binding and directives.
- Routing: Built-in routing support for creating SPAs with multiple views.
- Form Validation: Provides client-side form validation with built-in and custom validators.
- Testing: Designed with testing in mind, providing tools for unit and end-to-end testing.
Installation and Setup
Using a CDN
- Include AngularJS:
- Add the following script tag to your HTML file to include AngularJS from a CDN:
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js"></script>
- Add the following script tag to your HTML file to include AngularJS from a CDN:
Using npm
- Install AngularJS:
- Open your terminal and run:
npm install angular
- Open your terminal and run:
- Include AngularJS in Your Project:
- Import AngularJS in your JavaScript file:
import angular from 'angular';
- Import AngularJS in your JavaScript file:
Basic Concepts
Module
- Creating a Module:
- Define an AngularJS module using the
angular.module
method:const app = angular.module('myApp', []);
- Define an AngularJS module using the
Controller
- Creating a Controller:
- Define a controller within your module:
app.controller('MainController', function($scope) {
$scope.message = 'Hello, AngularJS!';
});
- Define a controller within your module:
- Binding the Controller to the View:
- Use the
ng-controller
directive in your HTML:<div ng-app="myApp" ng-controller="MainController">
{{ message }}
</div>
- Use the
Directives
- Built-In Directives:
- Use built-in directives like
ng-model
andng-repeat
:<input type="text" ng-model="name">
<p>Hello, {{ name }}!</p>
<ul>
<li ng-repeat=“item in items”>{{ item }}</li>
</ul>
- Use built-in directives like
- Custom Directives:
- Create custom directives to encapsulate reusable components:
app.directive('myDirective', function() {
return {
template: '<h1>My Custom Directive</h1>'
};
});
- Create custom directives to encapsulate reusable components:
Advanced Features
Services
- Creating a Service:
- Define a service to share data and functionality across the app:
app.service('myService', function() {
this.sayHello = function() {
return 'Hello from the service!';
};
});
- Define a service to share data and functionality across the app:
- Using a Service:
- Inject and use the service in a controller:
app.controller('MainController', function($scope, myService) {
$scope.greeting = myService.sayHello();
});
- Inject and use the service in a controller:
Routing
- Setting Up Routing:
- Include the
angular-route
module and configure routes:<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular-route.min.js"></script>
const app = angular.module('myApp', ['ngRoute']);
app.config(function($routeProvider) {
$routeProvider
.when(‘/’, {
templateUrl: ‘home.html’,
controller: ‘HomeController’
})
.when(‘/about’, {
templateUrl: ‘about.html’,
controller: ‘AboutController’
})
.otherwise({
redirectTo: ‘/’
});
});
- Include the
Form Validation
- Using Form Validation:
- Add validation to forms using AngularJS directives:
<form name="myForm">
<input type="email" name="email" ng-model="user.email" required>
<span ng-show="myForm.email.$error.required">Email is required.</span>
<span ng-show="myForm.email.$error.email">Invalid email address.</span>
<button type="submit" ng-disabled="myForm.$invalid">Submit</button>
</form>
- Add validation to forms using AngularJS directives:
Testing
Unit Testing
- Setting Up Testing Environment:
- Use tools like Jasmine and Karma for unit testing AngularJS applications.
- Install necessary packages:
npm install jasmine-core karma karma-jasmine karma-chrome-launcher --save-dev
- Writing Tests:
- Write unit tests for your controllers, services, and directives:
describe('MainController', function() {
beforeEach(module('myApp'));
let $controller, $rootScope;
beforeEach(inject(function(_$controller_, _$rootScope_) {
$controller = _$controller_;
$rootScope = _$rootScope_;
}));it(‘should initialize with a message’, function() {
let $scope = $rootScope.$new();
let controller = $controller(‘MainController’, { $scope: $scope });
expect($scope.message).toBe(‘Hello, AngularJS!’);
});
});
- Write unit tests for your controllers, services, and directives:
End-to-End Testing
- Setting Up Protractor:
- Use Protractor for end-to-end testing:
npm install protractor --save-dev
- Initialize Protractor configuration:
npx protractor --version
npx protractor init
- Use Protractor for end-to-end testing:
- Writing E2E Tests:
- Write E2E tests to simulate user interactions:
describe('My AngularJS App', function() {
it('should display the correct message', function() {
browser.get('http://localhost:8000');
let message = element(by.binding('message'));
expect(message.getText()).toEqual('Hello, AngularJS!');
});
});
- Write E2E tests to simulate user interactions:
Tips for Effective Use
- Modularize Your Code:
- Break your application into smaller, reusable modules for better organization and maintainability.
- Use
angular.module
to define and import modules.
- Leverage Built-In Directives:
- Use AngularJS’s powerful built-in directives to reduce the amount of code you need to write.
- Follow Best Practices:
- Follow AngularJS best practices for code structure, naming conventions, and component design.
- Optimize Performance:
- Minimize the use of watchers and avoid deep watch where possible.
- Use one-time bindings for static content.
- Stay Updated:
- Regularly check for updates and new features in AngularJS to take advantage of improvements and security patches.
- Use Angular CLI:
- Although primarily for Angular, the Angular CLI can help streamline your development workflow and manage dependencies.
Conclusion
AngularJS is a powerful and flexible framework for building dynamic single-page applications. Its robust features like two-way data binding, dependency injection, and directives make it an excellent choice for developers looking to create interactive web applications. By following this guide, you can set up, configure, and optimize your AngularJS development environment to take full advantage of its capabilities. Explore its features, leverage powerful tools, and follow best practices to ensure a productive and efficient development experience.